MongoDB
Masking a MongoDB database is essential for maintaining data security, ensuring compliance, and reducing risks associated with handling sensitive information. Unlike traditional relational databases, MongoDB’s document-based structure allows for flexible and scalable data storage, often containing personal, financial, or proprietary business information.
Without proper data masking, organizations face increased exposure to data breaches, unauthorized access, and regulatory penalties. By replacing sensitive values with anonymized or obfuscated data, masking helps protect personally identifiable information (PII) and other confidential records while preserving data usability for development, testing, and analytics.
For industries governed by strict data privacy regulations such as GDPR, HIPAA, or PCI-DSS, masking MongoDB databases ensures that non-production environments remain secure without compromising business operations. Implementing effective masking strategies with Runtime allows organizations to maintain data integrity, enhance security, and confidently work with realistic yet de-identified datasets.
MongoDB, Runtime and JSONPath
To mask fields in your database, you’ll need to define them using JSONPath. JSONPath provides a structured way to locate and reference specific fields within your data. This flexibility allows you to mask fields at various levels of your data hierarchy.
In the example below, you can see a demo database structure. To mask the field firstName, you can simply specify it as firstName
or use its full JSONPath: $.firstName
. Both approaches will correctly identify the field.
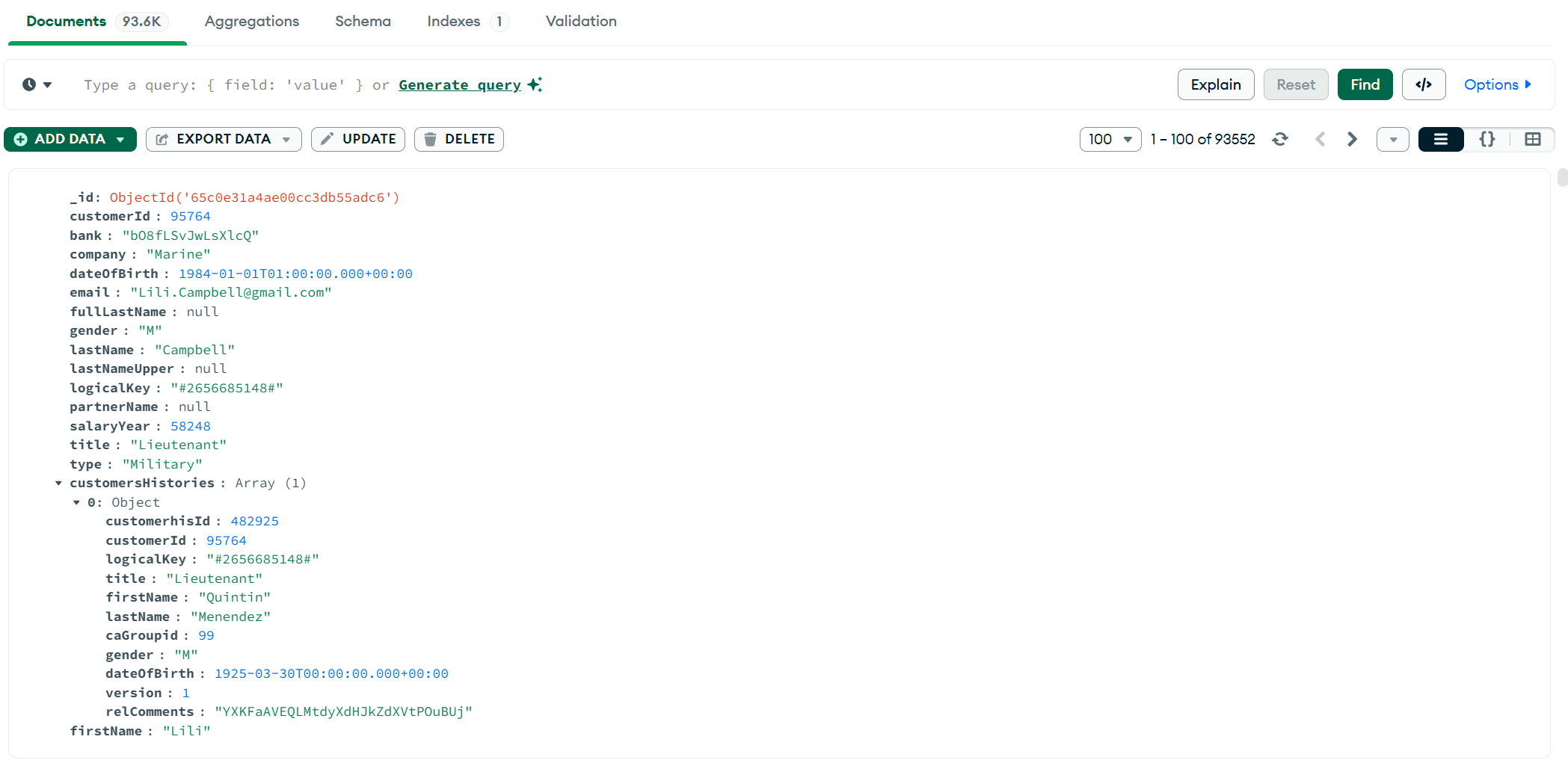
For more complex structures, such as arrays, you’ll need to use the JSONPath to navigate to the nested fields. For instance, consider an array named customerHistories that contains multiple fields. To mask the firstName field within this array, the JSONPath would be $.customerHistories.firstName
. This ensures that the masking process targets the correct field within the array.
By using JSONPath, you can accurately specify which fields to mask, regardless of their location within the document structure. This provides a precise and efficient method for ensuring sensitive data is securely managed.
Key JSONPath Operators
Operator | Description | Example | Explanation |
$ | Root node | $ | Selects the entire document |
. | Child node | $.customerId | Retrieves customer id’s e.g. 95764 |
.. | Recursive descent | $..dateOfBirth | Retrieves all dateOfBirth values |
[*] | Wildcard (all elements) | $.customersHistories[*].title | Retrieves all titles in customersHistories |
[index] | Specific index | $.customersHistories[0] | Retrieves the first record from customerHistories array |
['key'] | Specific key | $.['email'] | Retrieves values from email field, e.g. "datprof@gmail.com" |
Building a MongoDB Application
In this section, we will guide you through the process of building a custom application within DATPROF Runtime to effectively mask sensitive data in a MongoDB database. With the ability to use JSONPath for precise data targeting, you can configure masking rules to meet specific privacy and compliance requirements.
Before you can begin building a MongoDB application, you'll need to set up a group and an environment. Detailed instructions on this process can be found in the Groups and Environments section of the Runtime documentation.
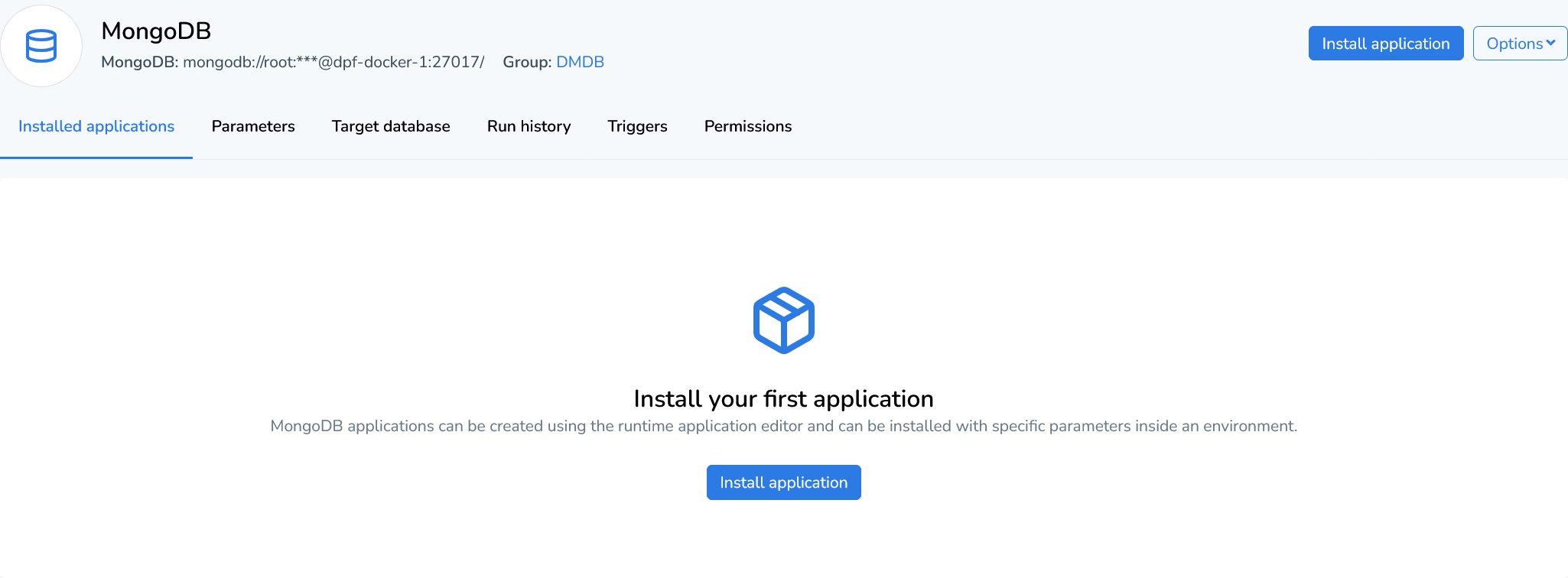
After pressing “Install Application”, you’ll be greeted by the following screen:
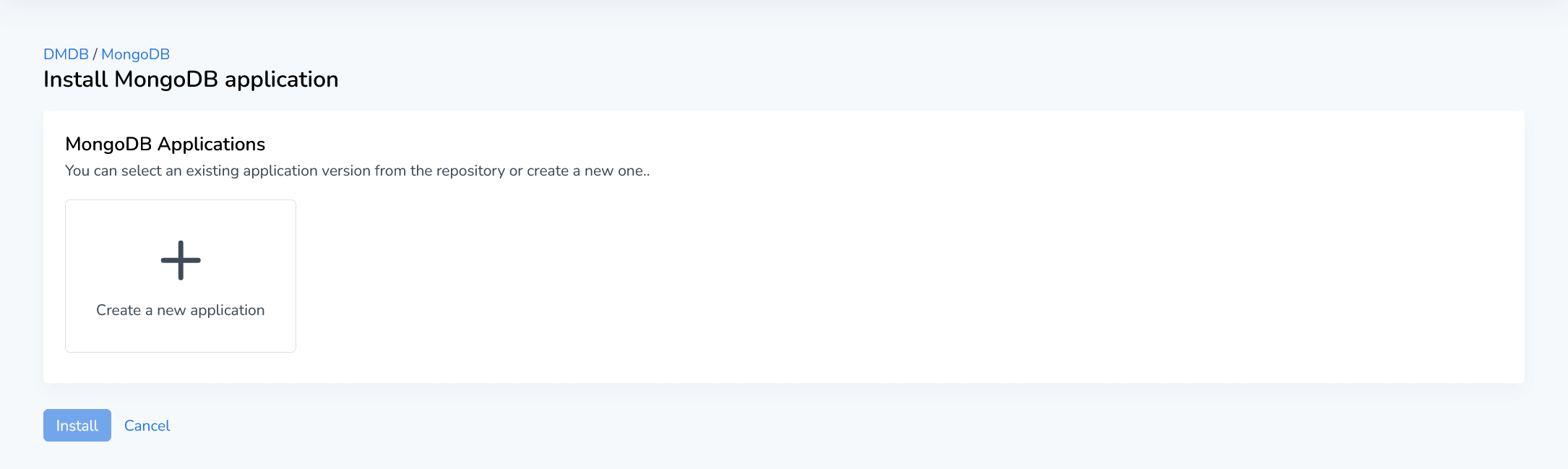
Press “Create a new application” to begin building your first application!
Application Editor
The interface of the Application editor is quite intuitive, but we’ll walk you through the key features to ensure you get the most out of it. Start by entering the following information:
Name: Enter a unique and descriptive name for your application. This name will be used to identify your application within the DATPROF Runtime interface.
Version: Specify the version of the application you’re creating. Versioning helps you manage updates and track changes to your application over time.
Description: Provide a brief yet informative description of your application. This should outline its purpose, key features, and any relevant details that can help users understand its function.
Once you’ve entered the application details, you’re ready to start building your application and configuring it to mask your MongoDB database!
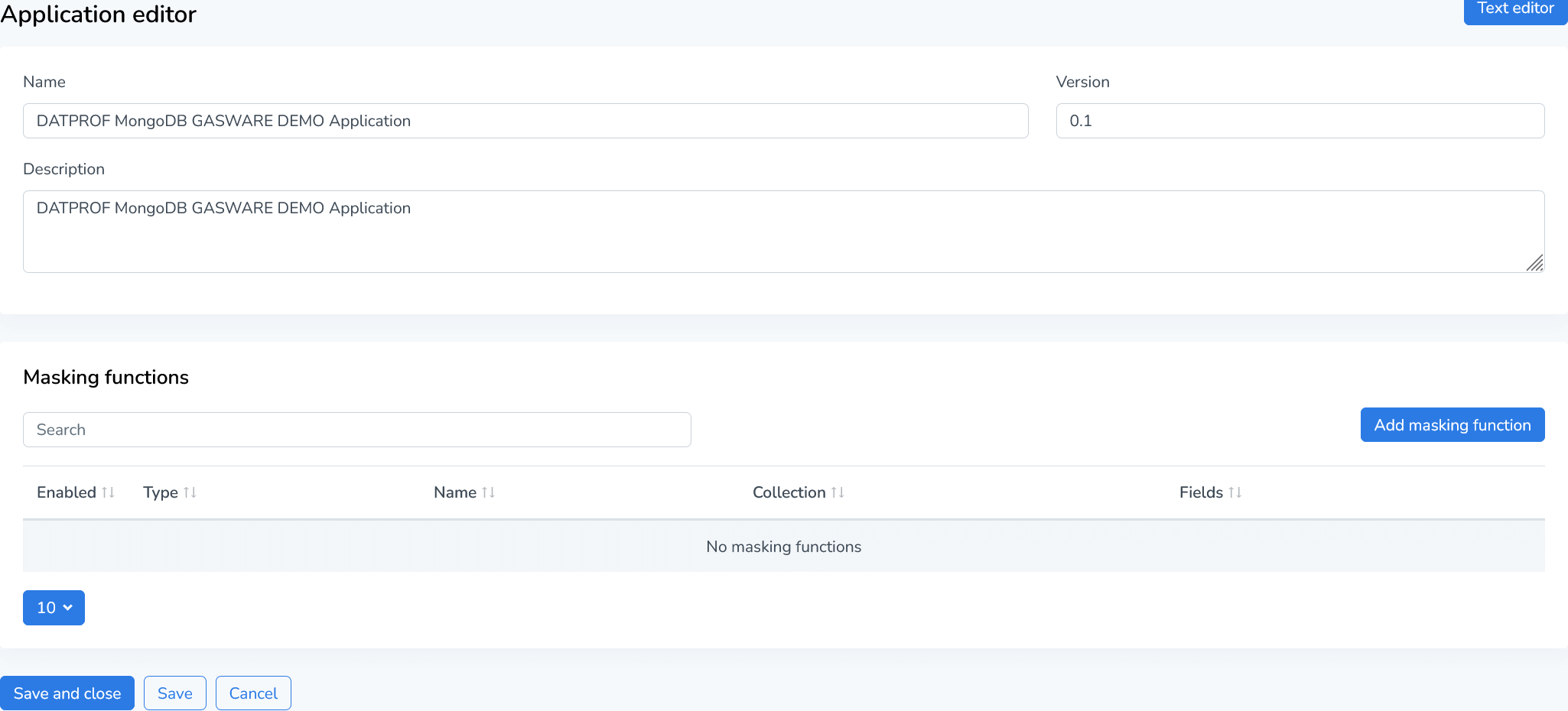
Masking Functions
DATPROF Runtime supports over 40 masking functions for MongoDB. Masking functions provide powerful and flexible ways to anonymize sensitive data within MongoDB databases. These functions allow you to apply a wide range of masking techniques, such as character replacement, data encryption, or randomization, to ensure that sensitive information is properly protected while maintaining the integrity and usability of the dataset. Whether you’re masking personal identifiers (PII), financial data, or other confidential information, DATPROF Runtime’s masking functions enable fine-grained control over how data is transformed.
This section will introduce you to the available masking functions for MongoDB.
Masking
The following data masking functions are available in Runtime for MongoDB:
Masking Functions | Description |
---|---|
Constant value | Creates a constant value in the generated dataset that is identical for every field. I.e. inputting MyFavoriteValue here will generate ‘MyFavoriteValue’ for every field in the resulting field’s dataset. |
Date/time modifier | This function will change the existing date to a fixed day in the same month. Or to a fixed day in the first month of the same year. With this change, in most cases the new values remain functionally viable. |
Custom expression | The JavaScript custom expression should resolve to a value or contain a function that returns a value. |
Value lookup | With this function the replacement value will be obtained from a lookup collection or translation collection. |
Blank | This function will NULL the selected field(s). |
Shuffle | The shuffle function ensures that all values in the selected field(s) are randomly mixed, like shuffling a deck of cards. The data distribution remains the same, which will ensure that the same values in the former situation, also obtain the same new values. A normal shuffle is a shuffle without selecting multiple fields. |
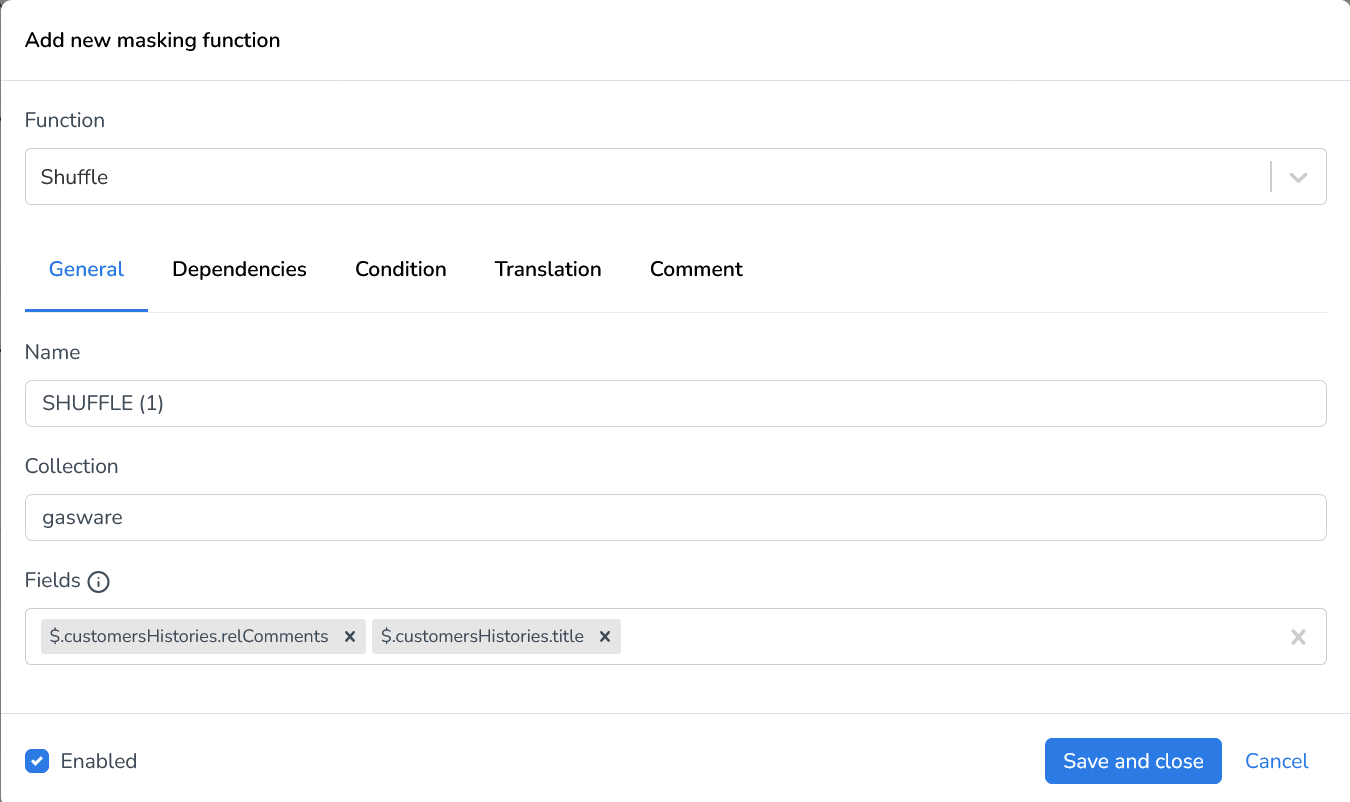
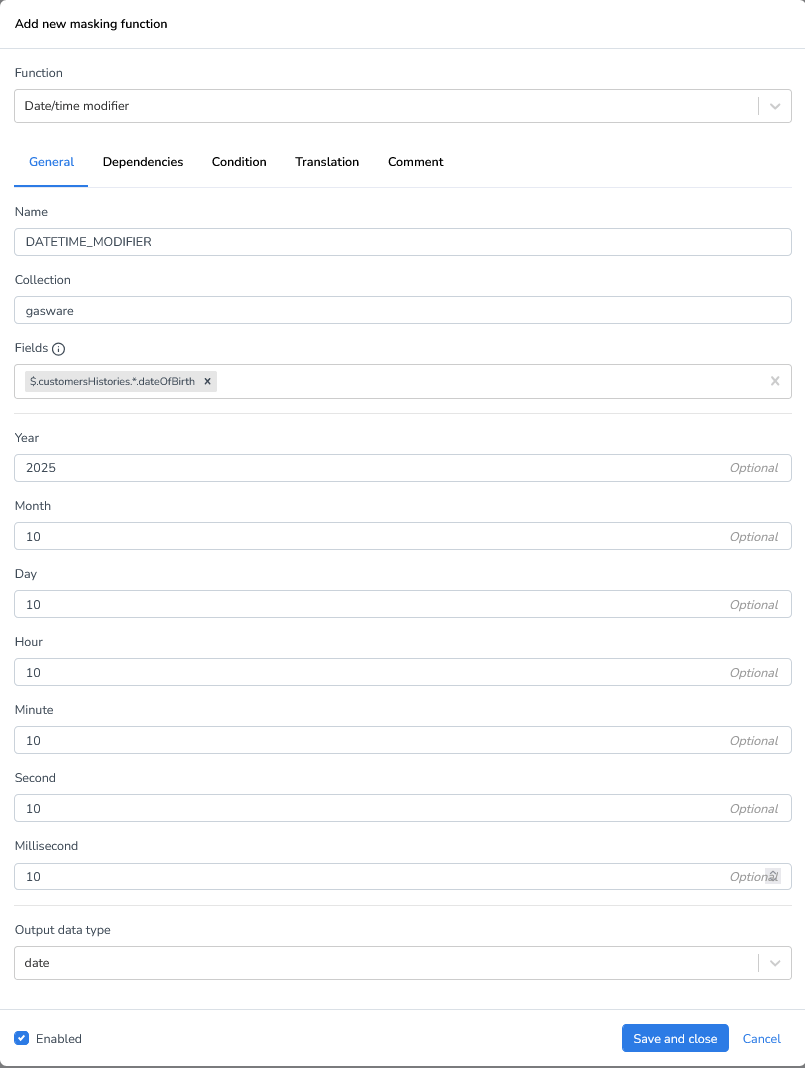
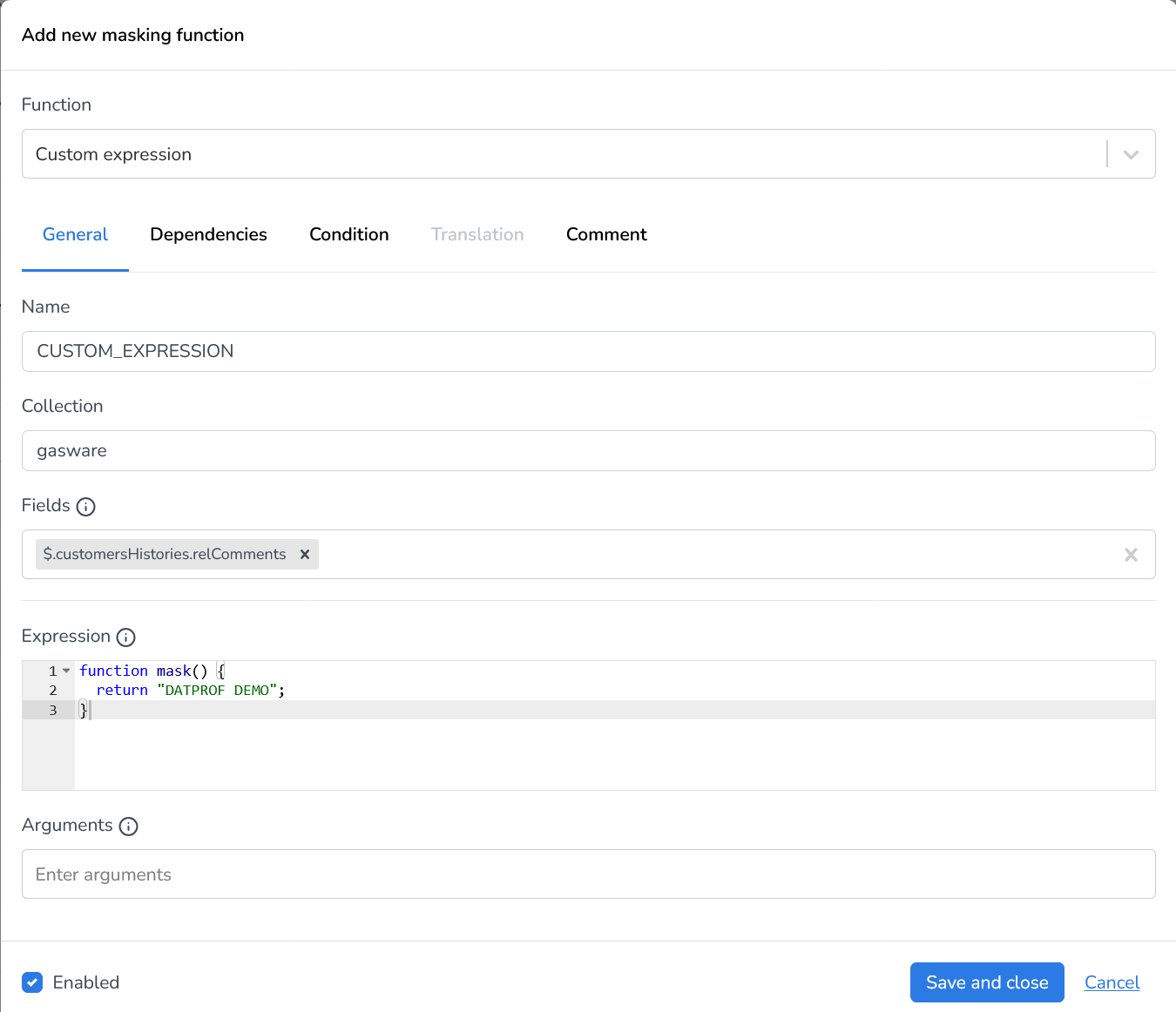
Basic Generators
The following basic generator functions are available in Runtime for MongoDB:
Basic Generators | Description |
---|---|
Random date/time | Generates a random Date/Time value per field between a specified minimum and maximum datetime that corresponds with the underlying field’s datatype. |
Sequential date/time | Identical to the Random Date/Time generator except that this generator creates a sequential datetime for every field. Supplying a maximum datetime is optional. A number to increment the starting date is required, and can only accept whole numbers. Any unit of time to increment by can be chosen, from seconds to years. |
Random decimal number | Generates a random decimal number between a supplied minimum and maximum value for every field. Using the Scale setting the decimal accuracy can be defined. For instance, a generator with a minimum value of 0 and a maximum value of 1000 using a scale of 4 might generate 132.4202. |
Random whole number | Generates a random integer between a supplied minimum and maximum value for every field. |
Sequential whole number | Generates a sequential number for every field that starts at a specified value and increments by the step value per field. Additionally, you can define a Padding for your generated integers. This is a set number that will be affixed to the generated integer. For example, using a padding of 3, and a start of 8 with a step of 2 will generate the following: 008 → 010 → 012 |
Random string | Generates a random string of lower- and uppercase letters for every field. The minimum and maximum length for strings can be defined. |
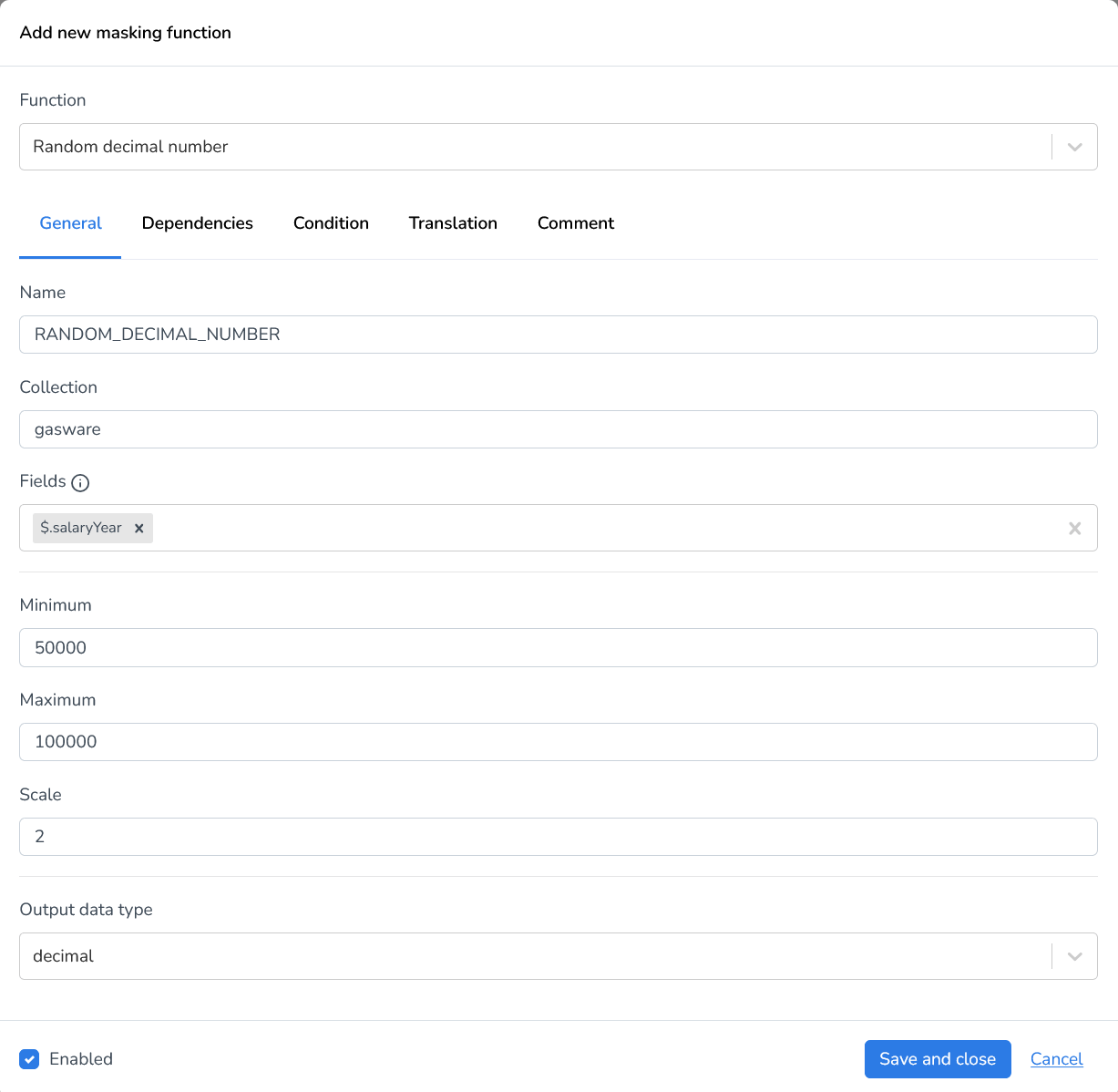
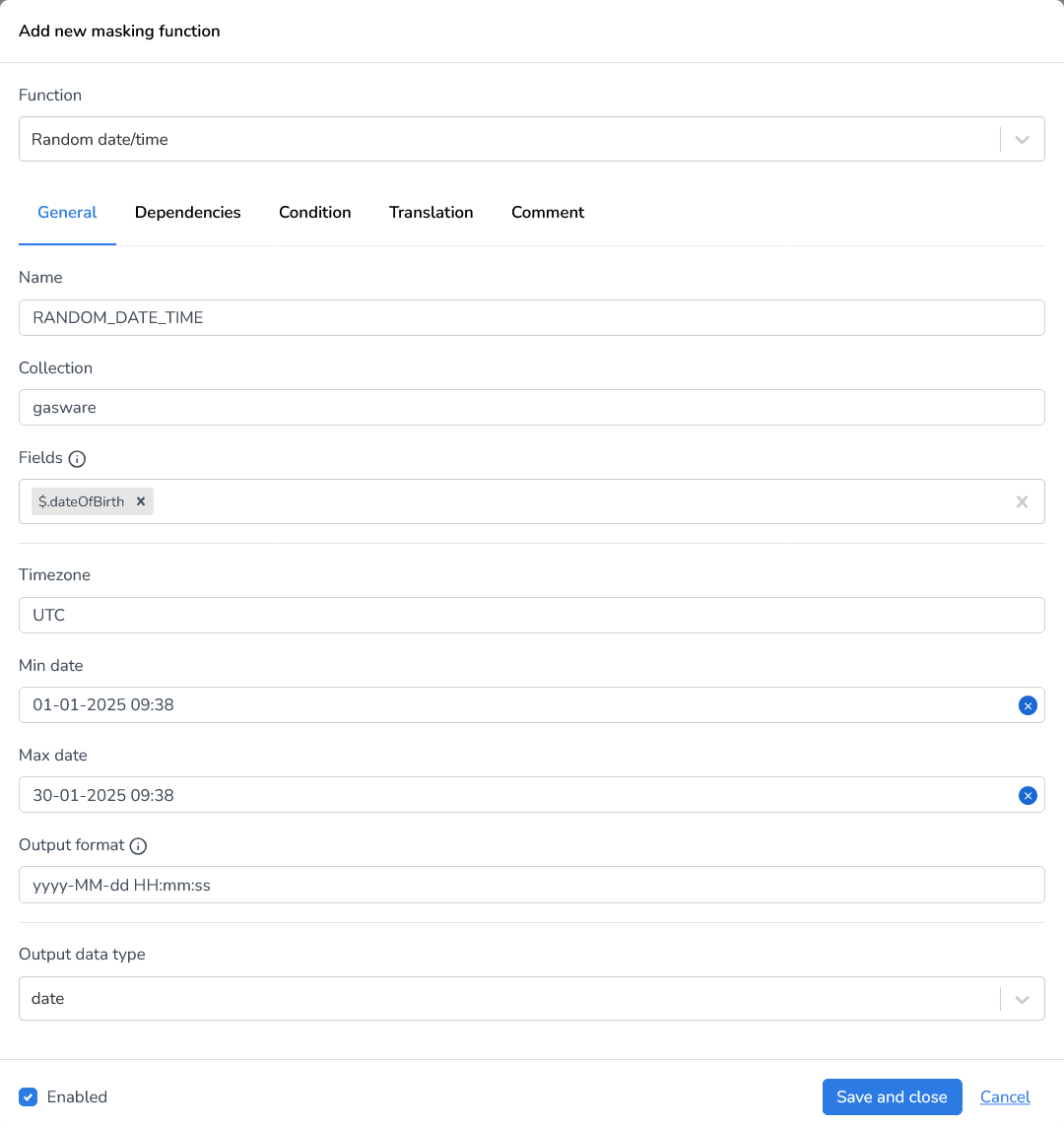
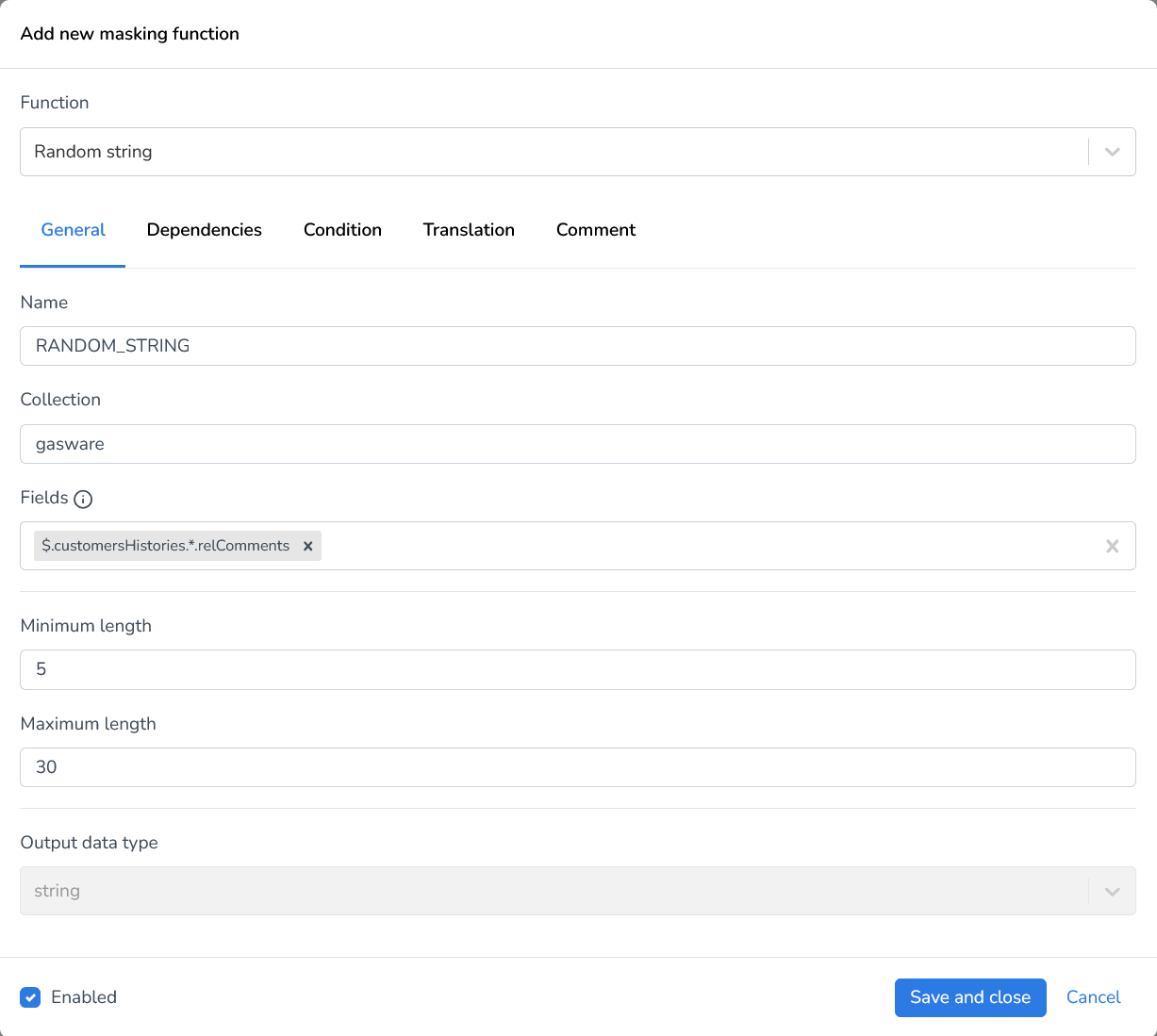
Business Generators
The following business generator functions are available in Runtime for MongoDB:
Business Generators | Description |
---|---|
Credit card account number | Generates a random credit card account number. Using Issuer(s), you must define one or multiple issuers to determine which syntax the generated account numbers adhere to. |
IBAN (International Bank Account Number) | Generates a valid IBAN number for every field. Using Country Code(s) you can specify which country codes you’d like your resulting IBAN codes to use. |
SSN (US Social Security Number) | Generates a SSN for every field. You can specify how you want to separate your resulting numbers. You can choose one of the following formats:
|
A-Number/GBA Number (Dutch township security number) | Generates a 10 digit GBA number per field. |
BSN Number (Dutch citizen service number) | Generates a valid Dutch social security number per field. |
Job | Generates a profession for every field. Using the Language(s) you can specify which language(s) you want your resulting job names generated in. |
Military rank | Generates a military rank name for every field. Using Department(s) you can specify which branches of the armed forces you’d like to include in your resulting dataset. |
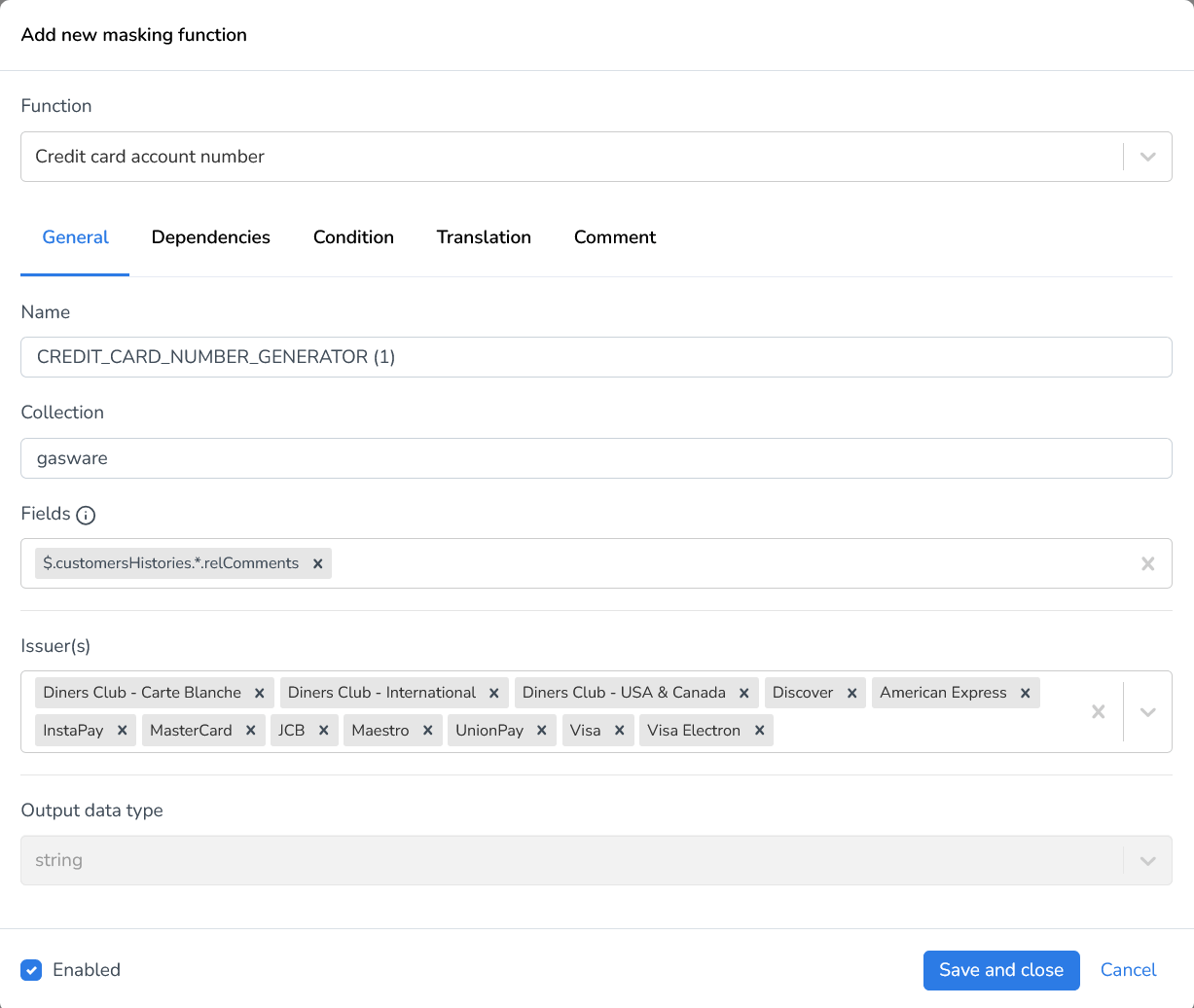

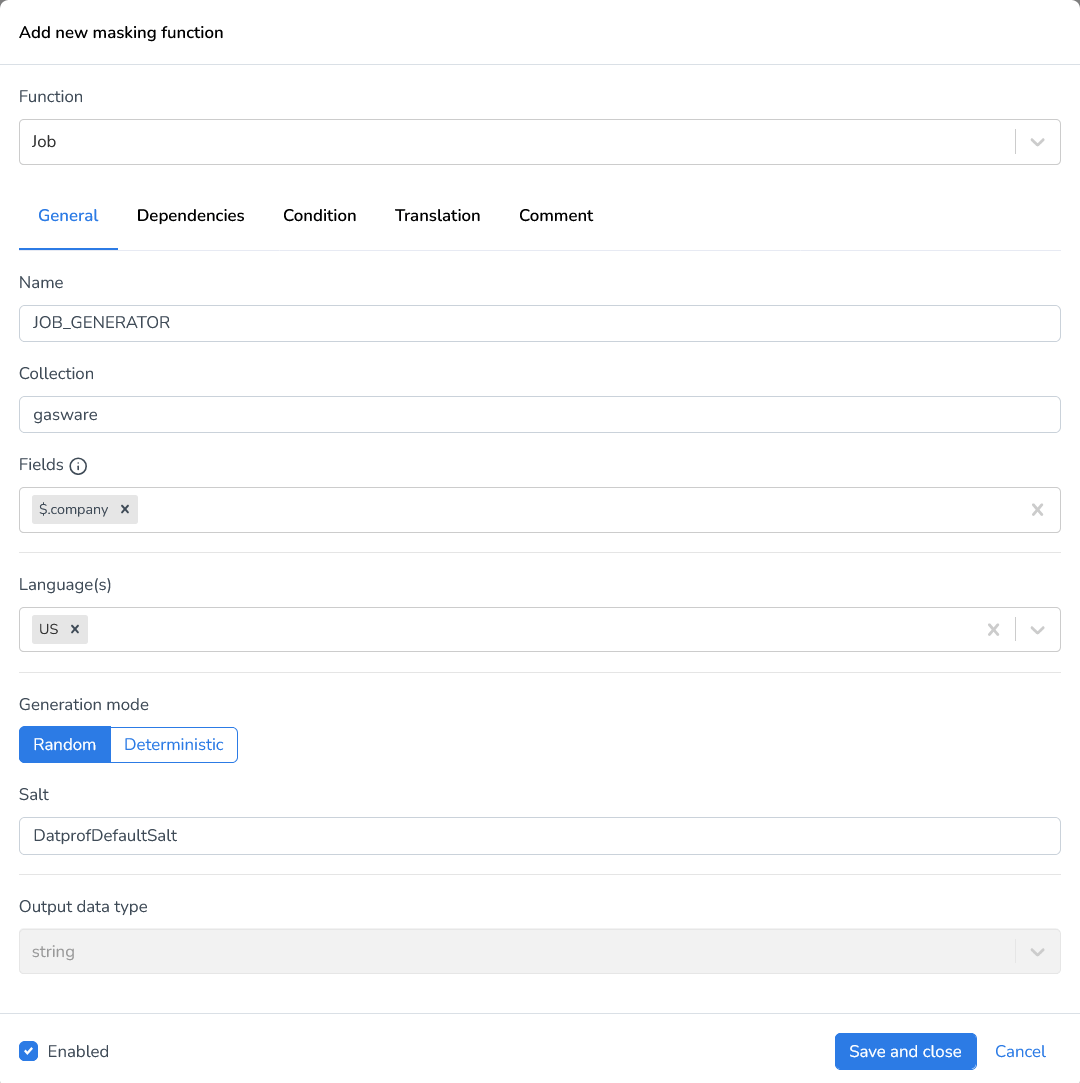
Advanced Generators
The following advanced generator functions are available in Runtime for MongoDB:
Advanced Generators | Description |
---|---|
Regular expression | Generates values based upon a regular expression. The syntax for the regular expression used in Runtime/Privacy is specific to the package we use, so please refer to the specifications here. |
Name Generators
The following name generator functions are available in Runtime for MongoDB:
Name Generators | Description |
---|---|
Brand | The Brand generator creates a unique brand name for each field in your dataset. It is particularly useful when working with data that requires distinct and realistic brand names. |
Color code | Generates a color hexcode (Both three-digit shorthand and six-digit full length hexcodes) per field. |
Color | Generates a random color per field. (Ex. Baby Blue, Sky Blue, Soft White) |
Two letter country code | Generates a random country code per field either in a 2 letter country code. |
Three letter country code | Generates a random country code per field either in a 4 letter country code. |
Currency code | Generates a three letter currency code for every field. |
Currency symbol | Generates a currency symbol for every field. |
Dinosaur | The Dinosaur generator generates a Dinosaur name for each field in your dataset. |
Genre | Generates a media genre for every field. |
User agent | Generates user agents per field, for example: Mozilla/4.0 (compatible; MSIE 5.13; Mac_PowerPC), Opera/8.53 (Windows NT 5.2; U; en). |
First name | The First name generator creates a unique first name for each field in your dataset. It is particularly useful when working with data that requires distinct and realistic first names. |
Full name | The Full name generator creates a unique full name for each field in your dataset. |
First name (female) | The First name (female) generator creates a unique female first name for each field in your dataset. |
First name (male) | The First name (male) generator creates a unique male first name for each field in your dataset. |
Last name | The Last name generator creates a unique last name for each field in your dataset. |
Random word | The Random word generator generates a random word for each field in your dataset. |
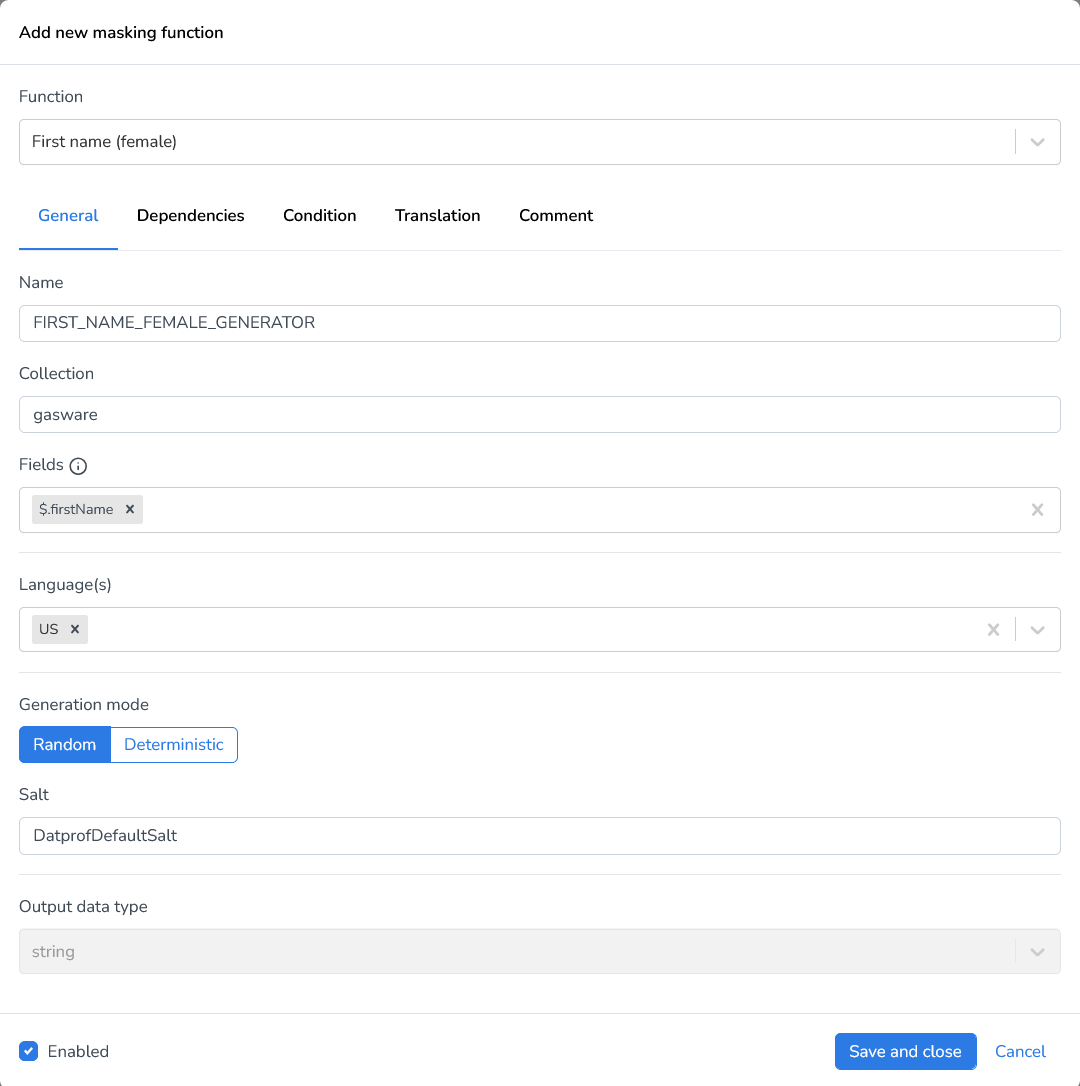
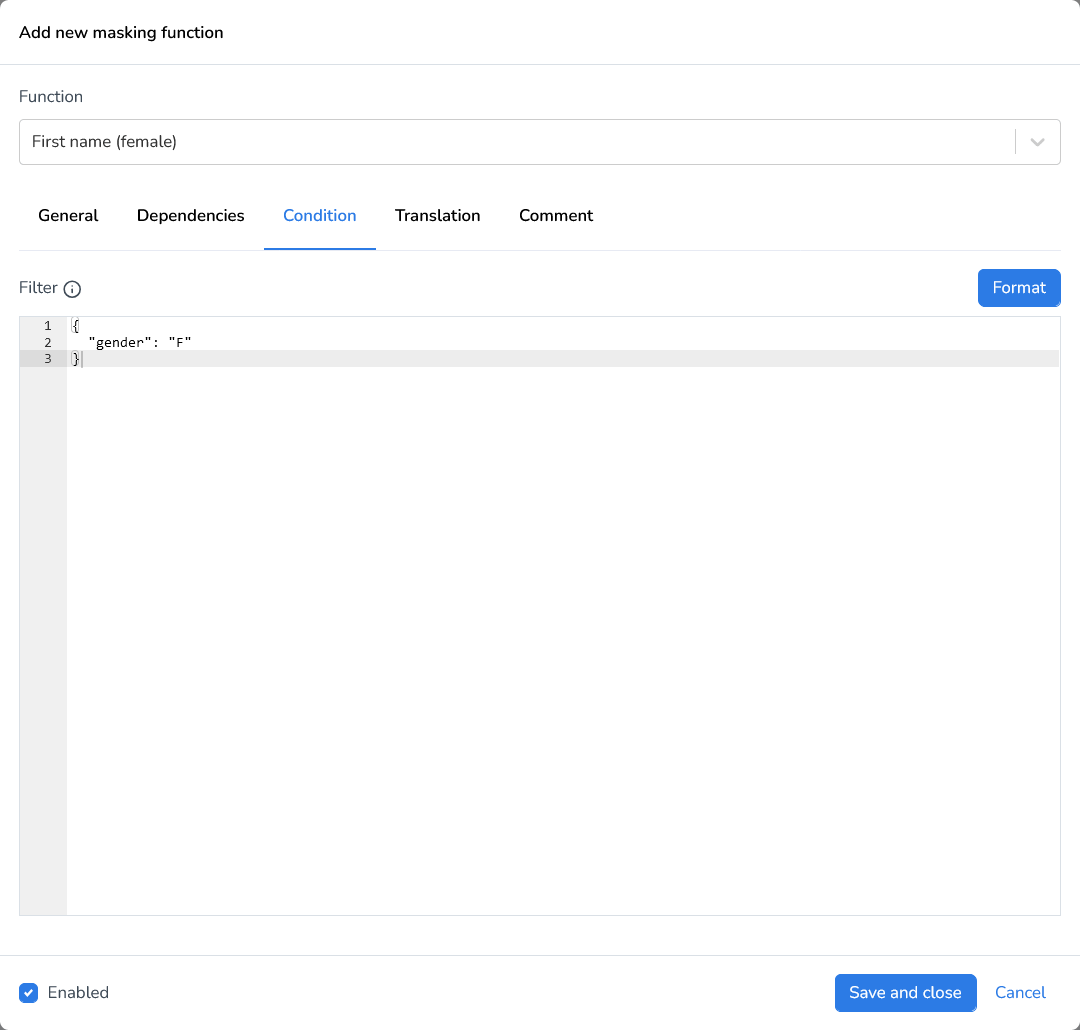
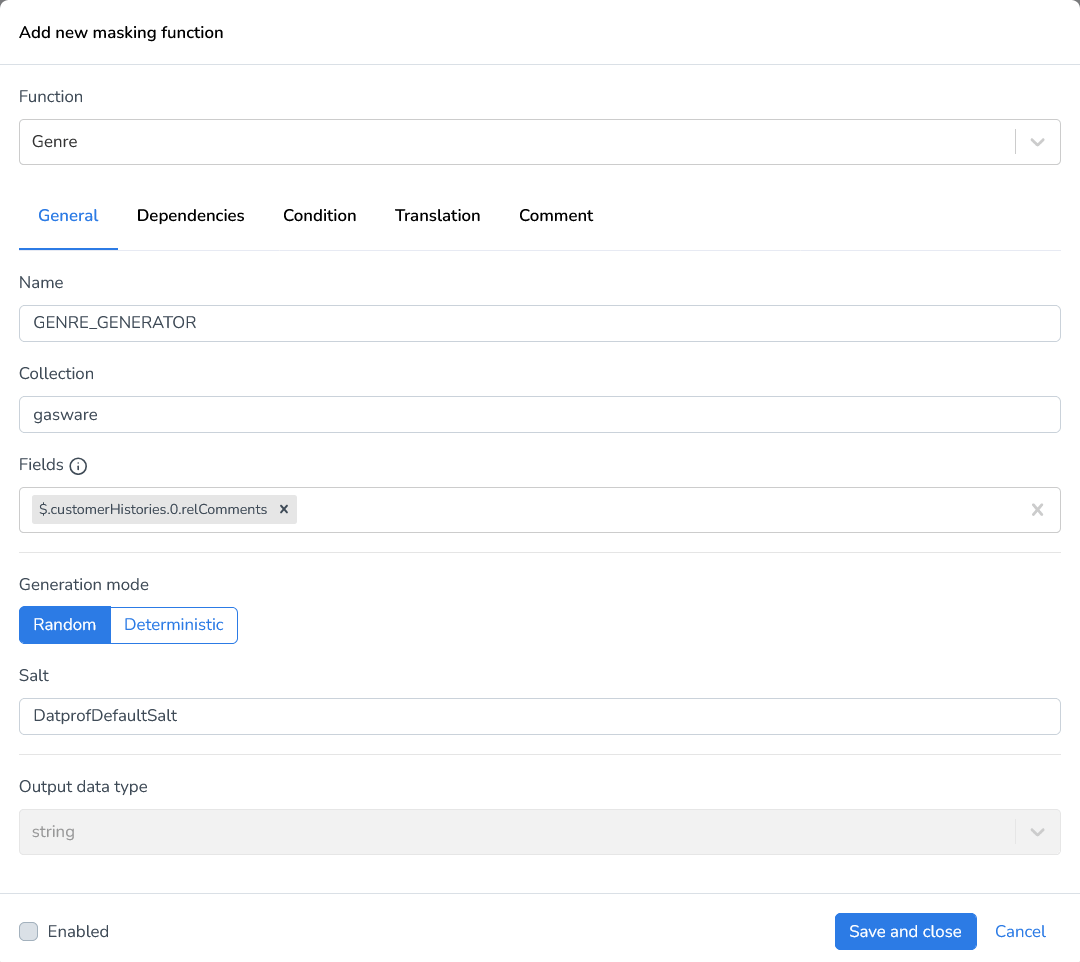
Location Generators
The following name generator functions are available in Runtime for MongoDB:
Location Generators | Description |
---|---|
City | Generates a random city name per field. Here, you can specify for which countries you’d like to generate random names using the Countries drop-down menu. |
Company | The Company generator generates a random company name for each field in your dataset. |
Country | Generates a random country name per field. The Language(s) option specifies in which language the country will be written. Multiple options can be enabled simultaneously. |
Street | Generates a random existing street name per field. The Countries option allows you to specify for which country street names will be generated. |
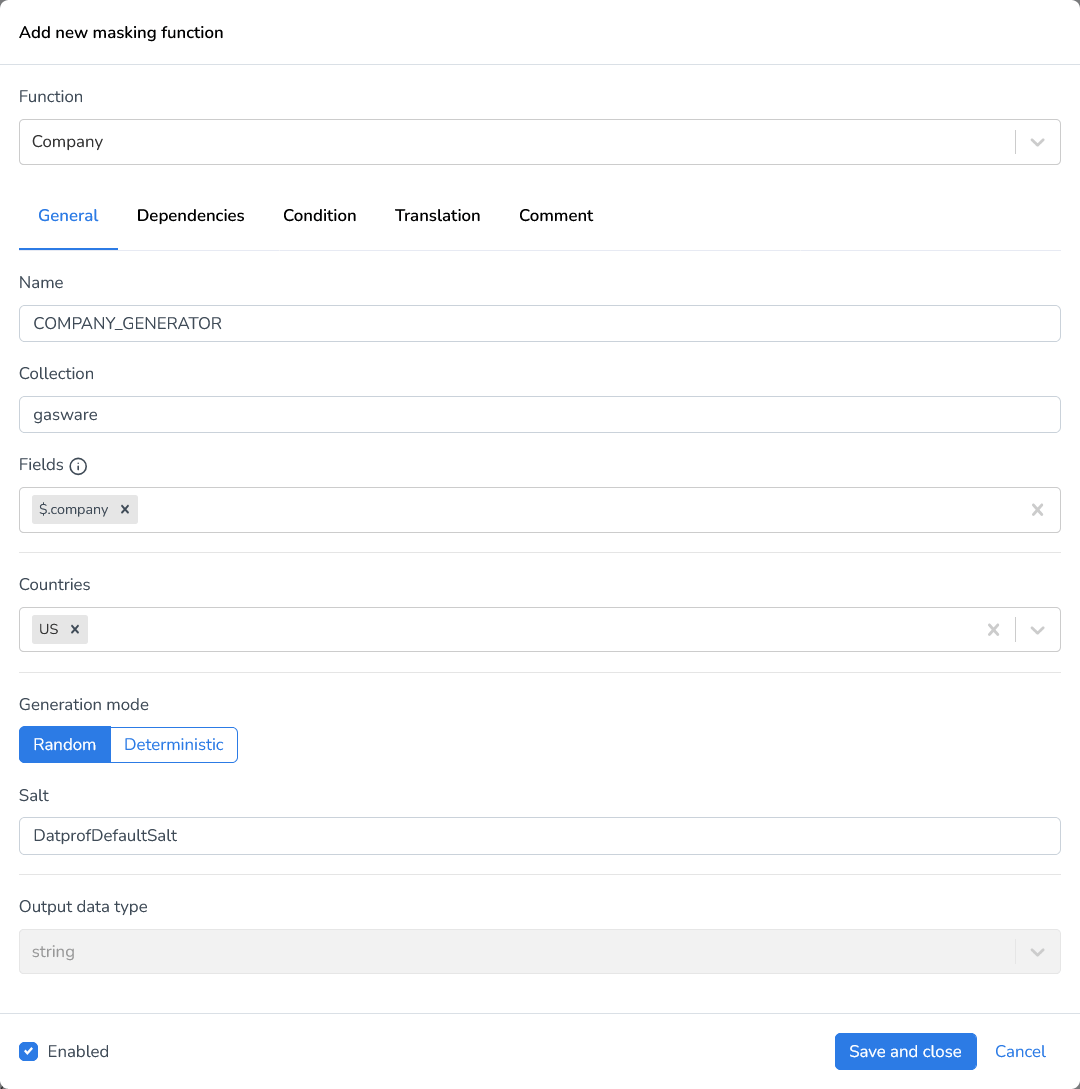
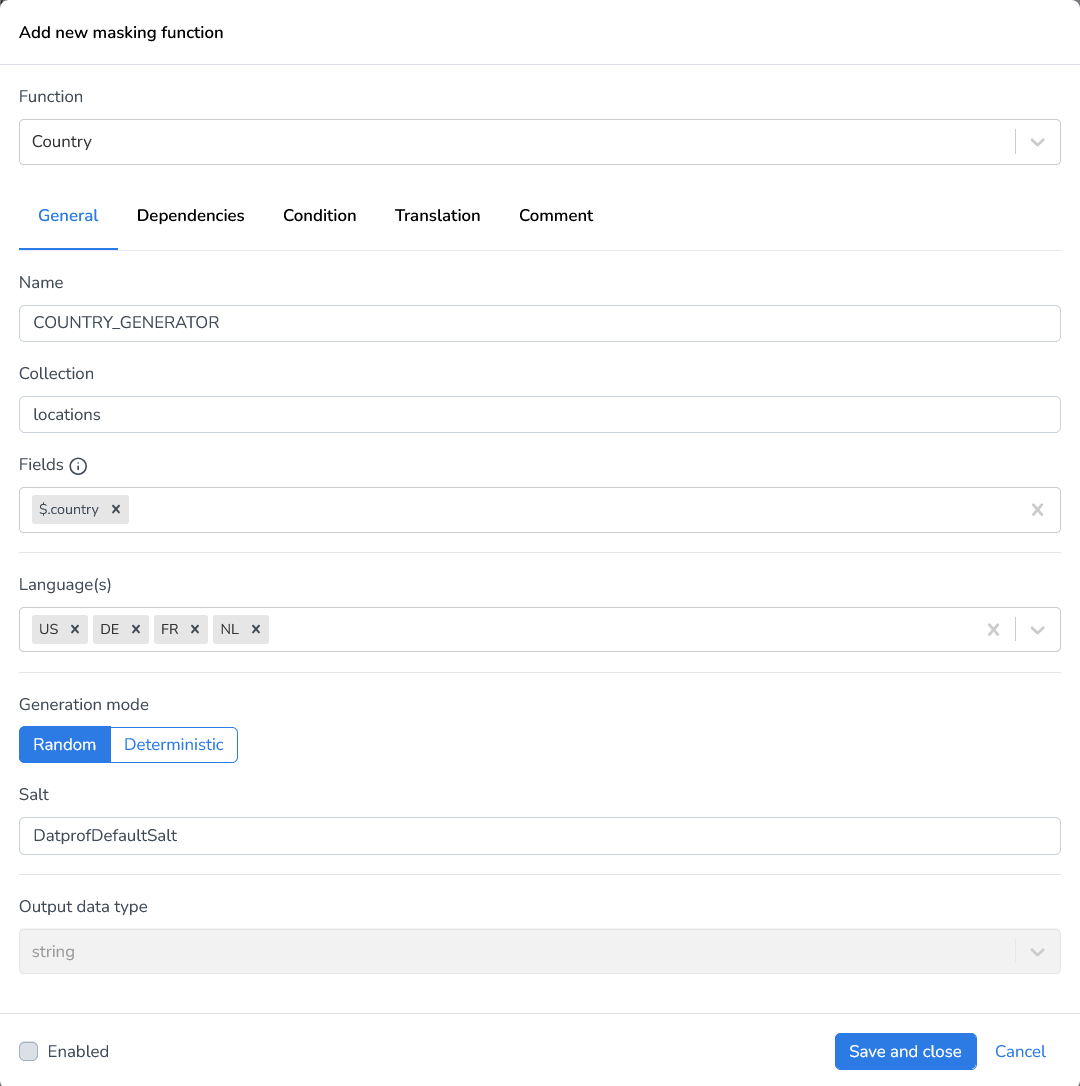
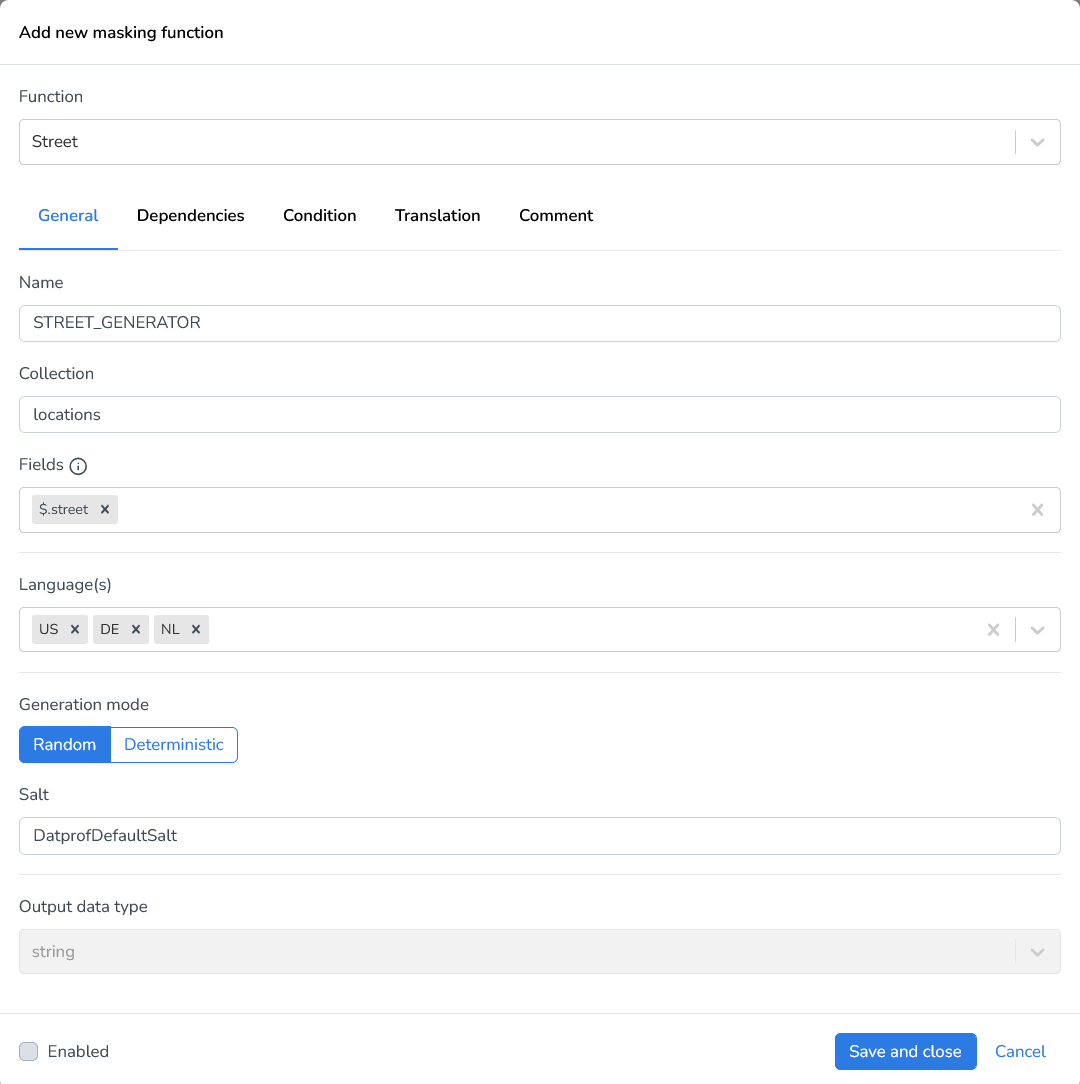
Dependencies
The Dependencies tab allows you to specify functions that the selected masking function depends on. This means the selected functions must complete first before this function is executed.
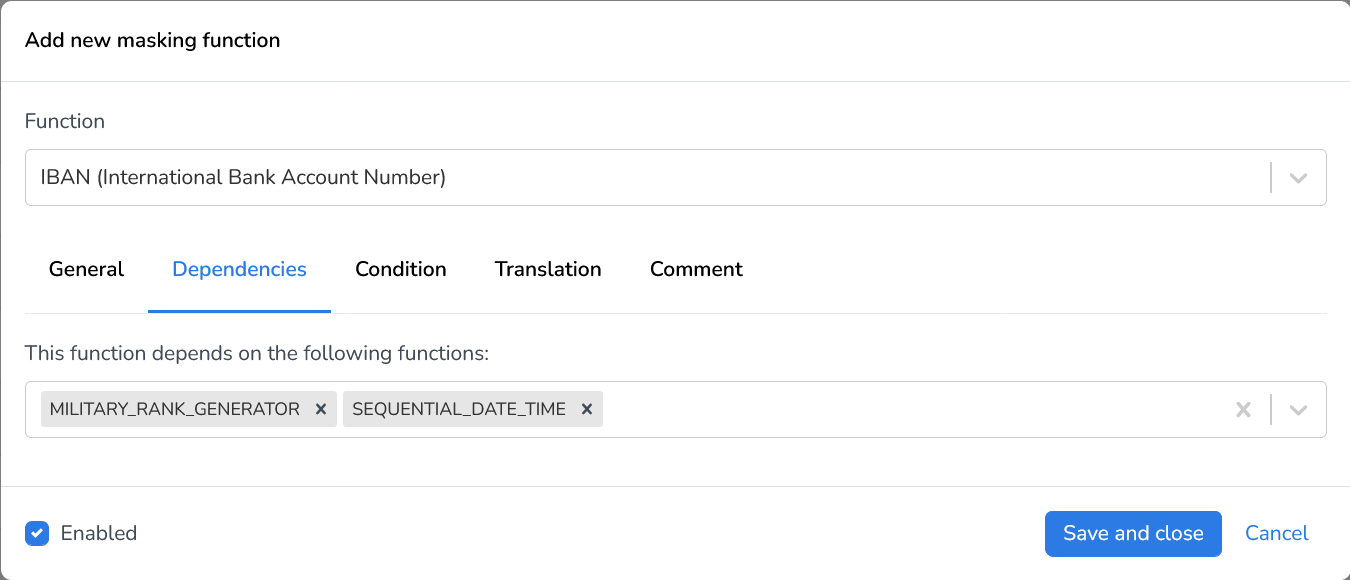
Condition
The Condition tab enables you to apply filters to the selected fields based on specific values. This allows you to define precise masking rules that apply only when certain conditions are met.
Format the filter as a MongoDB query predicate. (e.g. {last_name:'Smith'})
For example, in our demonstration, we’ll use the First Name (Female) generator to mask the selected field only when the gender field contains the value "F". This ensures that the masking function is applied selectively, preserving the integrity of other records while maintaining realistic and consistent data.
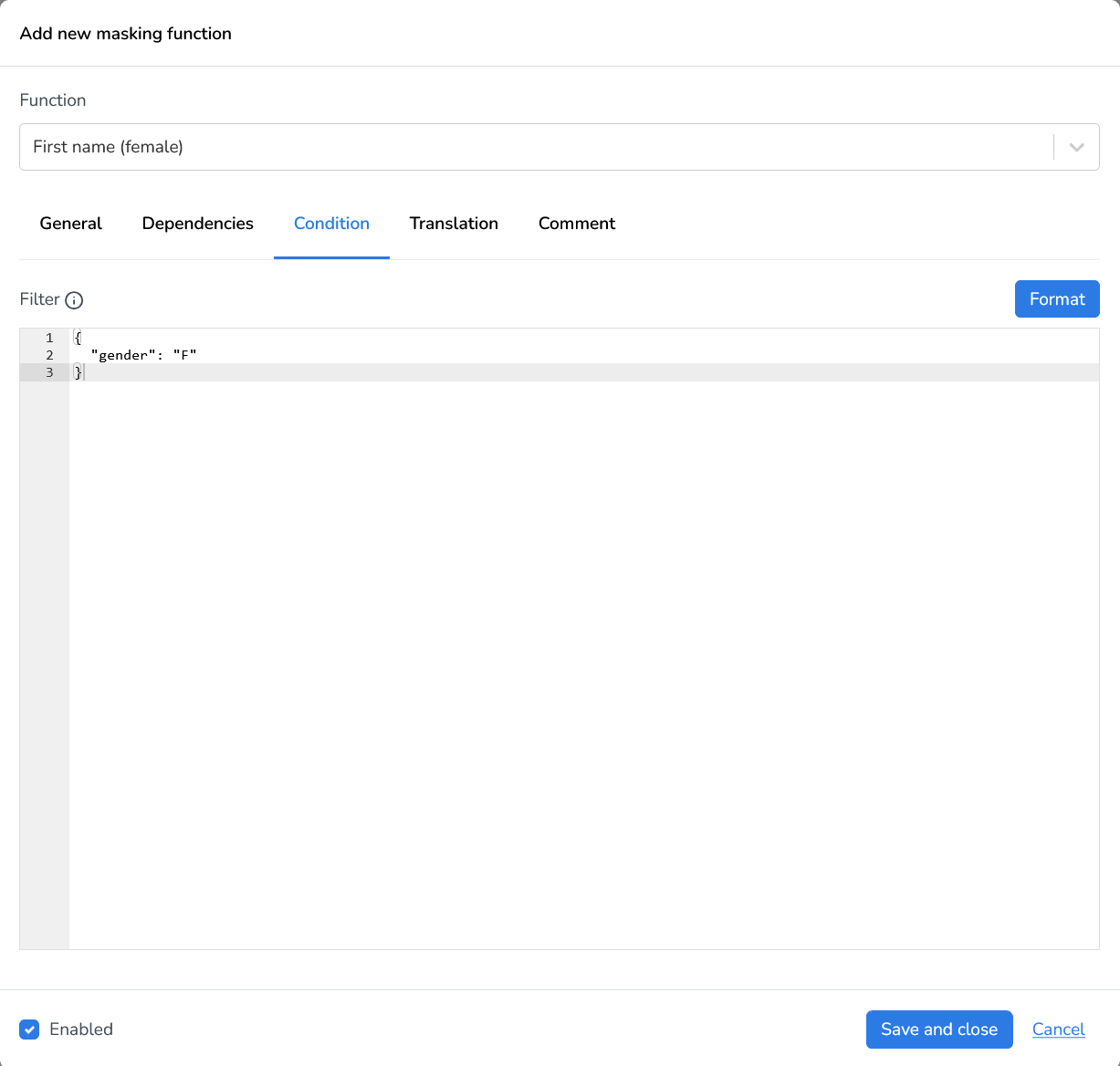
Translation
You can choose to store the result of the data masking function in a translation collection. This is particularly useful in implementing consistent data masking between collections. Translation collections store the old and the new value for each field value in the collection.
You can also use a ValueLookup function against a Translation collection when masking other collections or applications, effecting consistent masking across the Estate.
The Translation tab inside a masking function lets you create and name translation collections:
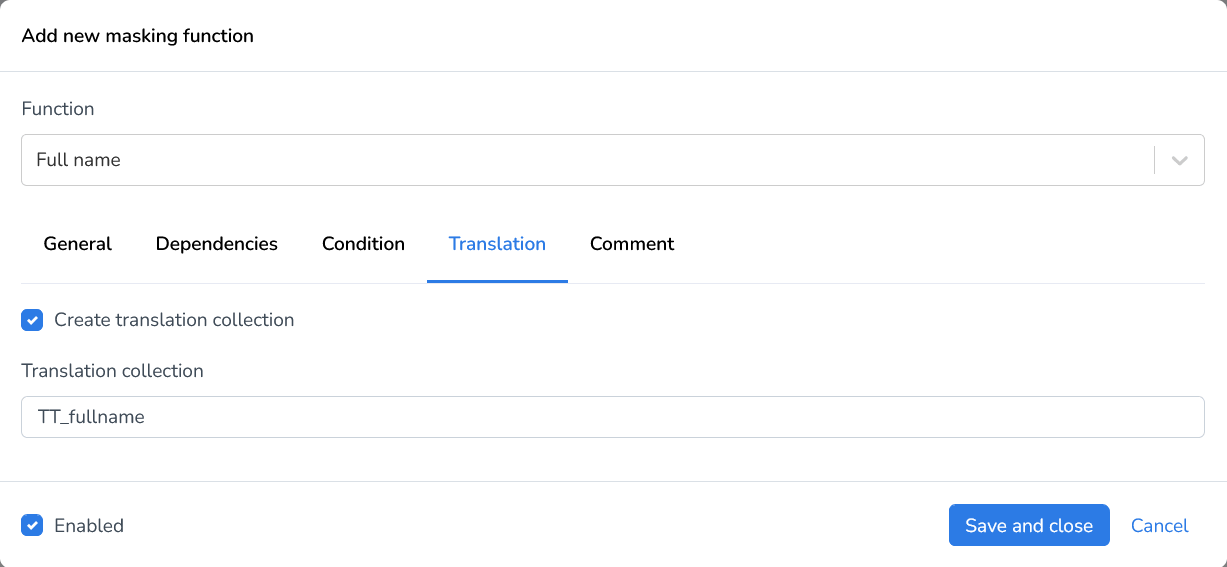
Output Datatypes
In MongoDB, output data types define the format of the data stored in documents and retrieved from the database. MongoDB’s flexible schema allows fields in a document to have various data types, ensuring optimal handling of diverse data structures.
Runtime supports multiple data types and can modify the data type of a field when necessary. You can specify the desired data type in your masking function to meet specific requirements. However, for certain functions, such as the IBAN generator function, the data type used may be predefined and cannot be changed.
Understanding these data types is essential for effectively managing, querying, and processing data within MongoDB and Runtime.
Datatype | Description | Example(s) |
---|---|---|
double | Represents a double-precision 64-bit floating-point number. This type is used for numerical values with decimals. | 3.14, -123.45 |
string | Stores text data as a sequence of characters. It is one of the most commonly used data types. | "DATPROF", "MongoDB" |
objectId | A unique identifier automatically generated for each document in a collection. It is a 12-byte value consisting of a timestamp and other unique information. | ObjectId("65c0e31a4ae00cc3db55adc6"). |
bool (Boolean) | Stores a binary value representing either true or false. It is used for logical fields. | true, false |
date | Represents a specific point in time, stored in milliseconds since the Unix epoch (January 1, 1970). | ISODate("2025-01-28T11:15:30Z") |
Int (Integer) | Stores 32-bit signed whole numbers, used for counting or indexing. | 42, -100 |
long | Stores 64-bit signed integers, allowing storage of larger whole numbers than int. | 9223372036854775807 |
decimal | Represents high-precision, 128-bit floating-point numbers, suitable for financial or other exact calculations. | Decimal128("1234.56789") |